Plugin Development - Custom Functions
Starting with v3.0, FoxVox introduced support for formula evaluations on variables. Formulas are created by preceding the variable value with the equals symbol (=):
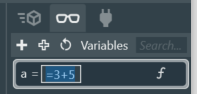
Setting variable a to the formula 3+5
This works fine, and illustrates how to set a variable to a formula, however in this case, it would be advised to simply assign the fixed value of 8, as the formula will add computational overhead within the library. There is no sense in adding extra processing overhead into the system when the result will always be the same.
A better use of a formula would be when a variable value is dependent on one or more other variables, and is subject to change:
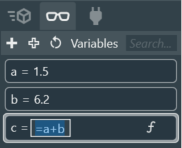
Setting variable c to the formula a+b
When referencing other variables inside formulas, remember variable names are case sensitive, and must match exactly. Once entered, the variable will display the resultant value along with a function indicator (F) that can be clicked to show/edit the underlying formula:
Variable showing function indicator
Built-in Functions
Functions from the .Net System.Math and System.String namespaces are built-in and available for use. These include common math operations such as Round, Power, Abs, etc. as well as standard string functions. Consult the Microsoft .Net documentation for more info. In addition, FoxVox provides a number of built-in operations itself. Here is a list of current functions and their arguments:
bool ContainsWord(this string str, string word, StringComparison stringComparison = StringComparison.InvariantCultureIgnoreCase) bool Equivalent(this string str, string s2, StringComparison stringComparison = StringComparison.InvariantCultureIgnoreCase) bool HasDigits(this string str) bool HasValue(this string str) bool IfAll(params bool[] ArgList) bool IfAny(params bool[] ArgList) bool IsASCIILetter(this char c) bool IsExecutable(this string file) bool IsLeapYear(int year) bool IsNullOrEmpty(this string str) bool IsNumeric(this string str, CultureInfo cultureInfo = null) bool Matches(this string str, string s2, StringComparison stringComparison = StringComparison.InvariantCultureIgnoreCase) bool ToBool(this object o) char? ToChar(this object value) DateTime AddDays(DateTime date, double amt) DateTime AddHours(DateTime date, double amt) DateTime AddMilliseconds(DateTime date, double amt) DateTime AddMinutes(DateTime date, double amt) DateTime AddMonths(DateTime date, int amt) DateTime AddSeconds(DateTime date, double amt) DateTime AddYears(DateTime date, int amt) DateTime Date(DateTime date) DateTime FromFileTime(long fileTime) DateTime GetDate(int year) DateTime GetDate(int year, int month) DateTime GetDate(int year, int month, int day) DateTime IfDate(bool cond, DateTime trueVal, DateTime falseVal) DateTime NextDayOfWeek(DateTime date, DayOfWeek weekDay) DateTime NextDayOfWeek(DateTime date, string WeekDay) DateTime NextFri(DateTime date) DateTime NextMon(DateTime date) DateTime NextSat(DateTime date) DateTime NextSun(DateTime date) DateTime NextThu(DateTime date) DateTime NextTue(DateTime date) DateTime NextWed(DateTime date) DateTime Now() DateTime ParseDate(string date) DateTime ToDate(this object value) DateTime Today() decimal ConvertToDec(this string value, decimal failValue = 0) decimal IfNum(bool cond, decimal trueVal, decimal falseVal) decimal Round(this decimal Amount, int Digits, decimal ToDec(this object value) double ConvertToDbl(this string value, double failValue = 0) double Round(this double Amount, int Digits, double Round(this float Amount, int Digits, double ToDbl(this object value) int ConvertToInt(this string value, int failValue = 0) int Day(DateTime date) int DayOfYear(DateTime date) int DeltaDeg(this int current, int target) int Hour(DateTime date) int Millisecond(DateTime date) int Minute(DateTime date) int Month(DateTime date) int Second(DateTime date) int SoundexDifference(this string data1, string data2) int ToInt(this decimal value) int Year(DateTime date) List<string> FirstWords(this string s, int count = 0) List<string> Merge(this IEnumerable<IEnumerable<string>> list) long FileTime(DateTime date) object If(bool cond, object trueVal, object falseVal) object IsNull(this object value, object altvalue) string AsNumber(this string str) string Combine(this string s1, string s2, string delimiter = " ") string DayOfWeek(DateTime date) string FormatAlphaNumeric(this string str, bool AllowWhiteSpace = false) string FormatDate(DateTime date, string format) string FormatNumeric(this string str, bool AllowDecimal = false) string IfStr(bool cond, string trueVal, string falseVal) string InsertSpaces(this string str) string Left(this string str, int length) string LongDate(DateTime date) string LongTime(DateTime date) string Prepend(this string s1, string s2, string delimiter = " ") string Remove(this string str, string remove, StringComparison stringComparison = StringComparison.CurrentCultureIgnoreCase) string RemoveConsecutiveWhiteSpace(this string str) string RemoveWhiteSpace(this string str) string RemoveWord(this string str, string word, StringComparison stringComparison = StringComparison.CurrentCultureIgnoreCase) string RemoveWords(this string str, params string[] words) string ReplaceFirst(this string s, string search, string replace) string ReplaceWord(this string str, string word, string replacement) string Right(this string str, int length) string Separate(this string str) string ShortDate(DateTime date) string ShortTime(DateTime date) string Soundex(this string s) string ToNATO(this string str) string ToStr(this object value)
One of the more commonly used functions is the If function. This provides conditional expressions and works just like it does in Excel. Here's an example:
=If(a+b>10, x, y)
If a + b is greater than 10, then value = x, otherwise value = y;
If statements can be used to compare numbers, strings, or other values. If the terms in the expression cannot be compared, an error message will appear. Expressions can be combined and nested within each other giving virtually unlimited possibilities.
Adding Custom Functions
FoxVox supports custom functions via Plugins. Plugins were originally designed to supply variables into FoxVox, and as such provide the perfect means to bring in custom functions as well. By default, public functions defined in the plugin are brought into FoxVox for use. Plugins do not need to have a polling method defined for these to work, either, although it is required for bringing in variables as described under Plugin Development. To disable evaluation functions on plugins, simply click the 'Disable Evaluation' option on the plugin:
Disable Evaluation on Plugins
Custom functions defined in the plugin can be used in variable formulas. Here's an example of a c# class that provides 2 custom functions, one to open a command prompt at an optional location, and another to get the triple value of a number:
using System.Diagnostics; namespace ClassLibrary1 { public static class MyFunctions { public static void Cmd(string StartDir = "") { Process.Start(new ProcessStartInfo { FileName = Path.Combine(Environment.SystemDirectory, "cmd.exe"), WorkingDirectory = StartDir, }); } public static double Triple(double val) { return val * 3; } } }
To use the 'Triple' function, a variable value would be set like this:
x = =Triple(1.5)
To use the Cmd function, you can open a window without a path:
x = =Cmd (parenthesis can be omitted since there is no argument)
OR
x = =Cmd("C:\Temp")
The Cmd function in the example doesn't return a value, but instead will simply open up a command window when evaluated. This is good for setting it on a variable used in an output so that the command window opens when you click a button or say a voice command. Of course you aren't limited to opening a command window. The method could call a game function or execute any custom code you want to create.
So there's the basics on the new formula evaluation support in FoxVox. Feel free to share your questions, comments, and experiences with this new feature.
Get FoxVox
FoxVox
Combination voice & key control application
Status | Released |
Category | Tool |
Author | foxster |
Tags | input, keyboard, macro, recognition, speech, talk, voice, voice-controlled |
Accessibility | Configurable controls |
More posts
- FoxVox Update v3.4.225 days ago
- FoxVox Update v3.4.144 days ago
- FoxVox Update v3.444 days ago
- Plugin Development - Voice Control60 days ago
- FoxVox Update v3.3.188 days ago
- FoxVox Update v3.397 days ago
- FoxVox Update v3.2.1Jan 24, 2025
- FoxVox Update v3.2Jan 24, 2025
- FoxVox Tutorial VideosJan 19, 2025
Leave a comment
Log in with itch.io to leave a comment.